Debug Formatter Helper ¶
The DebugFormatterHelper provides
functions to output debug information when running an external program, for
instance a process or HTTP request. For example, if you used it to output
the results of running figlet symfony
, it might output something like
this:
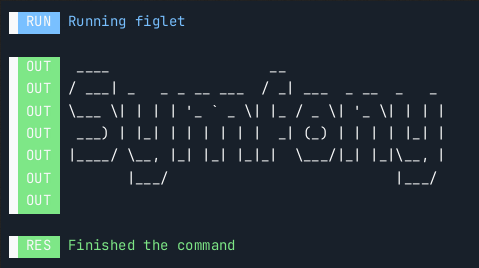
Using the debug_formatter ¶
The formatter is included in the default helper set and you can get it by calling getHelper():
1 |
$debugFormatter = $this->getHelper('debug_formatter');
|
The formatter accepts strings and returns a formatted string, which you then output to the console (or even log the information or do anything else).
All methods of this helper have an identifier as the first argument. This is a unique value for each program. This way, the helper can debug information for multiple programs at the same time. When using the Process component, you probably want to use spl_object_hash.
Tip
This information is often too verbose to be shown by default. You can use
verbosity levels to only show it when in
debugging mode (-vvv
).
Starting a Program ¶
As soon as you start a program, you can use start() to display information that the program is started:
1 2 3 4 5 6 7 8 9 |
// ...
$process = new Process(...);
$output->writeln($debugFormatter->start(
spl_object_hash($process),
'Some process description'
));
$process->run();
|
This will output:
1 |
RUN Some process description
|
You can tweak the prefix using the third argument:
1 2 3 4 5 6 7 |
$output->writeln($debugFormatter->start(
spl_object_hash($process),
'Some process description',
'STARTED'
));
// will output:
// STARTED Some process description
|
Output Progress Information ¶
Some programs give output while they are running. This information can be shown using progress():
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
use Symfony\Component\Process\Process;
// ...
$process = new Process(...);
$process->run(function ($type, $buffer) use ($output, $debugFormatter, $process) {
$output->writeln(
$debugFormatter->progress(
spl_object_hash($process),
$buffer,
Process::ERR === $type
)
);
});
// ...
|
In case of success, this will output:
1 |
OUT The output of the process
|
And this in case of failure:
1 |
ERR The output of the process
|
The third argument is a boolean which tells the function if the output is error
output or not. When true
, the output is considered error output.
The fourth and fifth argument allow you to override the prefix for the normal output and error output respectively.
Stopping a Program ¶
When a program is stopped, you can use stop() to notify this to the users:
1 2 3 4 5 6 7 8 |
// ...
$output->writeln(
$debugFormatter->stop(
spl_object_hash($process),
'Some command description',
$process->isSuccessful()
)
);
|
This will output:
1 |
RES Some command description
|
In case of failure, this will be in red and in case of success it will be green.
Using multiple Programs ¶
As said before, you can also use the helper to display more programs at the same time. Information about different programs will be shown in different colors, to make it clear which output belongs to which command.