How to Add "Remember Me" Login Functionality ¶
Once a user is authenticated, their credentials are typically stored in the
session. This means that when the session ends they will be logged out and
have to provide their login details again next time they wish to access the
application. You can allow users to choose to stay logged in for longer than
the session lasts using a cookie with the remember_me
firewall option:
-
YAML
YAML
-
XML
XML
-
PHP
PHP
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
remember_me:
secret: '%kernel.secret%' # required
lifetime: 604800 # 1 week in seconds
# by default, the feature is enabled by checking a
# checkbox in the login form (see below), uncomment the
# following line to always enable it.
#always_remember_me: true
|
The secret
option is the only required option and it is used to sign
the remember me cookie. It's common to use the kernel.secret
parameter,
which is defined using the APP_SECRET
environment variable.
After enabling the remember_me
system in the configuration, there are a
couple more things to do before remember me works correctly:
- Add an opt-in checkbox to activate remember me;オプトイン チェックボックスを追加して、記憶を有効にします。
- Use an authenticator that supports remember me;Remember Me をサポートするオーセンティケーターを使用してください。
- Optionally, configure how remember me cookies are stored and validated.必要に応じて、remember me Cookie の保存方法と検証方法を構成します。
After this, the remember me cookie will be created upon successful authentication. For some pages/actions, you can force a user to fully authenticate (i.e. not through a remember me cookie) for better security.
Note
The remember_me
setting contains many settings to configure the
cookie created by this feature. See Customizing the Remember Me Cookie
for a full description of these settings.
Activating the Remember Me System ¶
Using the remember me cookie is not always appropriate (e.g. you should not use it on a shared PC). This is why by default, Symfony requires your users to opt-in to the remember me system via a request parameter.
This request parameter is often set via a checkbox in the login form. This
checkbox must have a name of _remember_me
:
1 2 3 4 5 6 7 8 9 10 11 |
{# templates/security/login.html.twig #}
<form method="post">
{# ... your form fields #}
<label>
<input type="checkbox" name="_remember_me" checked/>
Keep me logged in
</label>
{# ... #}
</form>
|
Note
Optionally, you can configure a custom name for this checkbox using the
name
setting under the remember_me
section.
Always activating Remember Me ¶
Sometimes, you may wish to always activate the remember me system and not
allow users to opt-out. In these cases, you can use the
always_remember_me
setting:
-
YAML
YAML
-
XML
XML
-
PHP
PHP
1 2 3 4 5 6 7 8 9 10 11 |
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
remember_me:
secret: '%kernel.secret%'
# ...
always_remember_me: true
|
Now, no request parameter is checked and each successful authentication will produce a remember me cookie.
Add Remember Me Support to the Authenticator ¶
Not all authentication methods support remember me (e.g. HTTP Basic
authentication doesn't have support). An authenticator indicates support
using a RememberMeBadge
on the security passport.
After logging in, you can use the security profiler to see if this badge is present:
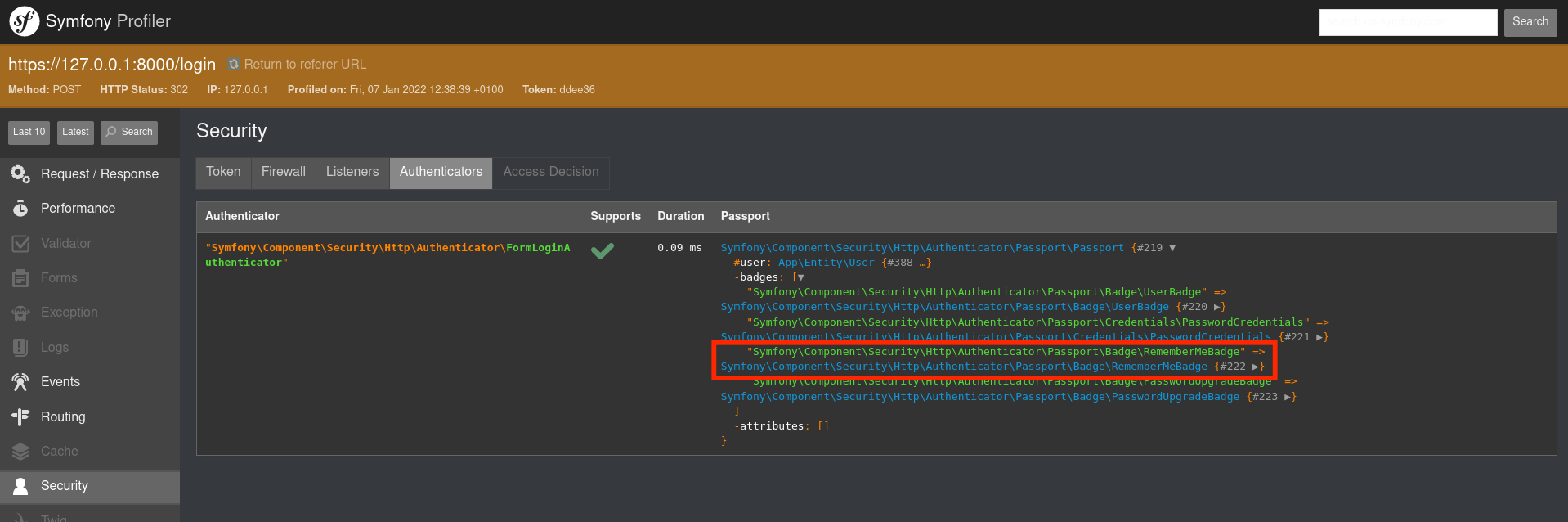
Without this badge, remember me will be not be activated (regardless of all other settings).
Add Remember Me Support to Custom Authenticators ¶
When you use a custom authenticator, you must add a RememberMeBadge
manually:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// src/Service/LoginAuthenticator.php
namespace App\Service;
// ...
use Symfony\Component\Security\Http\Authenticator\Passport\Badge\RememberMeBadge;
use Symfony\Component\Security\Http\Authenticator\Passport\Badge\UserBadge;
use Symfony\Component\Security\Http\Authenticator\Passport\Passport;
class LoginAuthenticator extends AbstractAuthenticator
{
public function authenticate(Request $request): Passport
{
// ...
return new Passport(
new UserBadge(...),
new PasswordCredentials(...),
[
new RememberMeBadge(),
]
);
}
}
|
Customize how Remember Me Tokens are Stored ¶
Remember me cookies contain a token that is used to verify the user's identity. As these tokens are long-lived, it is important to take precautions to allow invalidating any generated tokens.
Symfony provides two ways to validate remember me tokens:
- Signature based tokens
-
By default, the remember me cookie contains a signature based on
properties of the user. If the properties change, the signature changes
and already generated tokens are no longer considered valid. See
How to Add "Remember Me" Login Functionality for more information.
デフォルトでは、remember me cookie には、ユーザーのプロパティに基づく署名が含まれています。プロパティが変更されると、署名が変更され、既に生成されたトークンは有効と見なされなくなります。詳細については、「Remember Me」ログイン機能を追加する方法を参照してください。
- Persistent tokens
-
Persistent tokens store any generated token (e.g. in a database). This
allows you to invalidate tokens by changing the rows in the database.
See How to Add "Remember Me" Login Functionality for more information.
永続的なトークンは、生成されたトークンを (データベースなどに) 保存します。これにより、データベース内の行を変更してトークンを無効にすることができます。詳細については、「Remember Me」ログイン機能を追加する方法を参照してください。
Note
You can also write your own custom remember me handler by creating a
class that extends
AbstractRememberMeHandler
(or implements RememberMeHandlerInterface).
You can then configure this custom handler by configuring the service
ID in the service
option under remember_me
.
Using Signed Remember Me Tokens ¶
By default, remember me cookies contain a hash that is used to validate the cookie. This hash is computed based on configured signature properties.
These properties are always included in the hash:
- The user identifier (returned by
getUserIdentifier());ユーザー識別子 (getUserIdentifier() によって返されます);
- The expiration timestamp.有効期限のタイムスタンプ。
On top of these, you can configure custom properties using the
signature_properties
setting (defaults to password
). The properties
are fetched from the user object using the
PropertyAccess component (e.g. using
getUpdatedAt()
or a public $updatedAt
property when using
updatedAt
).
-
YAML
YAML
-
XML
XML
-
PHP
PHP
1 2 3 4 5 6 7 8 9 10 11 |
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
remember_me:
secret: '%kernel.secret%'
# ...
signature_properties: ['password', 'updatedAt']
|
In this example, the remember me cookie will no longer be considered valid
if the updatedAt
, password or user identifier for this user changes.
Tip
Signature properties allow for some advanced usages without having to
set-up storage for all remember me tokens. For instance, you can add a
forceReloginAt
field to your user and to the signature properties.
This way, you can invalidate all remember me tokens from a user by
changing this timestamp.
Storing Remember Me Tokens in the Database ¶
As remember me tokens are often long-lived, you might prefer to save them in a database to have full control over them. Symfony comes with support for persistent remember me tokens.
This implementation uses a remember me token provider for storing and retrieving the tokens from the database. The DoctrineBridge provides a token provider using Doctrine.
You can enable the doctrine token provider using the doctrine
setting:
-
YAML
YAML
-
XML
XML
-
PHP
PHP
1 2 3 4 5 6 7 8 9 10 11 12 |
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
remember_me:
secret: '%kernel.secret%'
# ...
token_provider:
doctrine: true
|
This also instructs Doctrine to create a table for the remember me tokens. If you use the DoctrineMigrationsBundle, you can create a new migration for this:
1 2 3 4 |
$ php bin/console doctrine:migrations:diff
# and optionally run the migrations locally
$ php bin/console doctrine:migrations:migrate
|
Otherwise, you can use the doctrine:schema:update
command:
1 2 3 4 5 |
# get the required SQL code
$ php bin/console doctrine:schema:update --dump-sql
# run the SQL in your DB client, or let the command run it for you
$ php bin/console doctrine:schema:update --force
|
Implementing a Custom Token Provider ¶
You can also create a custom token provider by creating a class that implements TokenProviderInterface.
Then, configure the service ID of your custom token provider as service
:
-
YAML
YAML
-
XML
XML
-
PHP
PHP
1 2 3 4 5 6 7 8 9 10 11 |
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
remember_me:
# ...
token_provider:
service: App\Security\RememberMe\CustomTokenProvider
|
Forcing the User to Re-Authenticate before Accessing certain Resources ¶
When the user returns to your site, they are authenticated automatically based on the information stored in the remember me cookie. This allows the user to access protected resources as if the user had actually authenticated upon visiting the site.
In some cases, however, you may want to force the user to actually re-authenticate before accessing certain resources. For example, you might not allow "remember me" users to change their password. You can do this by leveraging a few special "attributes":
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// src/Controller/AccountController.php
// ...
public function accountInfo(): Response
{
// allow any authenticated user - we don't care if they just
// logged in, or are logged in via a remember me cookie
$this->denyAccessUnlessGranted('IS_AUTHENTICATED_REMEMBERED');
// ...
}
public function resetPassword(): Response
{
// require the user to log in during *this* session
// if they were only logged in via a remember me cookie, they
// will be redirected to the login page
$this->denyAccessUnlessGranted('IS_AUTHENTICATED_FULLY');
// ...
}
|
Tip
There is also a IS_REMEMBERED
attribute that grants access only
when the user is authenticated via the remember me mechanism.
Customizing the Remember Me Cookie ¶
The remember_me
configuration contains many options to customize the
cookie created by the system:
name
(default value:REMEMBERME
)-
The name of the cookie used to keep the user logged in. If you enable the
remember_me
feature in several firewalls of the same application, make sure to choose a different name for the cookie of each firewall. Otherwise, you'll face lots of security related problems.ユーザーのログイン状態を維持するために使用される Cookie の名前。同じアプリケーションの複数のファイアウォールでremember_me 機能を有効にする場合は、各ファイアウォールの Cookie に異なる名前を選択してください。そうしないと、多くのセキュリティ関連の問題に直面することになります。 lifetime
(default value:31536000
i.e. 1 year in seconds)-
The number of seconds after which the cookie will be expired. This
defines the maximum time between two visits for the user to remain
authenticated.
Cookie の有効期限が切れるまでの秒数。これは、ユーザーが認証されたままになるための 2 回のアクセス間の最大時間を定義します。
path
(default value:/
)-
The path where the cookie associated with this feature is used. By default
the cookie will be applied to the entire website but you can restrict to a
specific section (e.g.
/forum
,/admin
).この機能に関連付けられた Cookie が使用されるパス。デフォルトでは、Cookie は Web サイト全体に適用されますが、特定のセクション (/forum、/admin など) に制限することができます。 domain
(default value:null
)-
The domain where the cookie associated with this feature is used. By default
cookies use the current domain obtained from
$_SERVER
.この機能に関連付けられた Cookie が使用されるドメイン。デフォルトでは、Cookie は $_SERVER から取得した現在のドメインを使用します。 secure
(default value:false
)-
If
true
, the cookie associated with this feature is sent to the user through an HTTPS secure connection.true の場合、この機能に関連付けられた Cookie が HTTPS セキュア接続を介してユーザーに送信されます。 httponly
(default value:true
)-
If
true
, the cookie associated with this feature is accessible only through the HTTP protocol. This means that the cookie won't be accessible by scripting languages, such as JavaScript.true の場合、この機能に関連付けられた Cookie には、HTTP プロトコルを介してのみアクセスできます。これは、JavaScript などのスクリプト言語から Cookie にアクセスできないことを意味します。 samesite
(default value:null
)-
If set to
strict
, the cookie associated with this feature will not be sent along with cross-site requests, even when following a regular link.厳密に設定すると、通常のリンクをたどった場合でも、この機能に関連付けられた Cookie はクロスサイト リクエストとともに送信されません。